javascript get file extension before upload with code example
Last Updated: 2023-02-15 10:35:43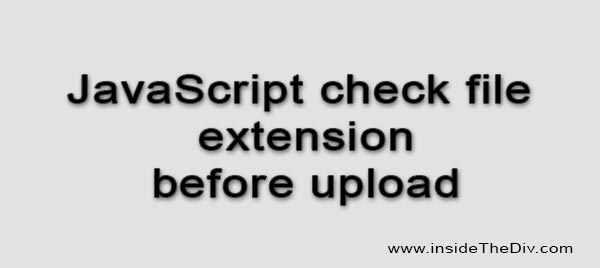
When uploading files to a server using a web application, it is important to validate the file type before the upload. Validating the file type ensures that the user uploads only the files that the application can handle, reducing the chances of security breaches and errors. In this blog post, we will discuss why and how to validate the file type before the upload using JavaScript, along with its advantages and disadvantages.
What is The File Type, Extension, or MIME?
A file type is a type of file format. A file extension is a set of characters added to the end of the filename to indicate the file type. MIME (Multipurpose Internet Mail Extensions) is a type of identifier that defines the format of files that are exchanged on the internet.
Why do we need to check the file extension before uploading?
Checking file types before uploading helps to prevent security breaches by ensuring that only the intended files are uploaded. For example, if an application only accepts image files, a user uploading a script file with malicious code could result in an exploit of the application. Checking the file type also helps to prevent errors by avoiding the uploading of incompatible file types.
JavaScript code to check file extension before upload
The following code demonstrates how to check the file type before uploading using JavaScript.
const fileInput = document.getElementById("file");
fileInput.addEventListener("change", (event) => {
const file = event.target.files[0];
const fileType = file["type"];
const validImageTypes = ["image/jpeg", "image/png", "image/gif"];
if (!validImageTypes.includes(fileType)) {
alert("Invalid file type. Please upload an image file.");
event.target.value = "";
}
});
The code above listens to the file input element for a change event. Once a change occurs, the code retrieves the file object and gets its type. It then compares the file type with an array of valid file types. If the file type is not in the valid file types array, the code shows an alert message and resets the file input element value.
How to validate the file extension of multiple files when they are uploaded simultaneously using JavaScript:
const fileInput = document.getElementById("file");
fileInput.addEventListener("change", (event) => {
const files = event.target.files;
for (let i = 0; i < files.length; i++) {
const fileType = files[i]["type"];
const validImageTypes = ["image/jpeg", "image/png", "image/gif"];
if (!validImageTypes.includes(fileType)) {
alert("Invalid file type. Please upload an image file.");
event.target.value = "";
return;
}
}
});
The codes above listen to the file input element for a change event. Once a change occurs, the code retrieves an array of file objects and loops through them. For each file, the code gets its type and compares it with an array of valid file types. If the file type is not in the valid file types array, the code shows an alert message and resets the file input element value. The return statement inside the loop ensures that only one error message is shown, even if multiple files have invalid types.
This updated code can be used to ensure that all files uploaded by the user are of the correct type, regardless of how many files are selected at once.
If you want to learn more about the input field validation you can read those posts, here I have explained with code examples how to validate a login form in javascript and how to validate a registration form in javascript. Also, you can learn how to validate a valid email in javascript.
Javascript validation only PNG format image is allow validation
When building a website or web application that allows users to upload images, it's important to ensure that only valid image files are accepted. One common requirement is to only allow PNG format images to be uploaded.
In JavaScript, you can perform file validation using the File object that represents the selected file. To validate that the file is a PNG image, you can check both the file type and the file extension.
The type property of the File object represents the MIME type of the file. For PNG images, the MIME type is image/png. You can check if the file type matches this using the === operator:
function isPng(file) {
return file.type === 'image/png';
}
However, some browsers may not correctly set the type property for image files, so it's also important to check the file extension. The name property of the File object includes the filename and extension. To check if the extension is .png, you can use the endsWith method of the String object:
function isPng(file) {
return file.type === 'image/png' && file.name.toLowerCase().endsWith('.png');
}
The toLowerCase method is used to make the extension check case-insensitive.
Once you have this function, you can use it to validate the selected file when the user submits the form or selects the file. If the file is not a PNG image, you can prevent the form from submitting and display an error message to the user:
const fileInput = document.getElementById('my-file-input');
const form = document.getElementById('my-form');
form.addEventListener('submit', (event) => {
if (!isPng(fileInput.files[0])) {
event.preventDefault();
alert('Please select a PNG image file.');
}
});
By performing this simple validation in JavaScript, you can ensure that your web application only accepts valid PNG images, providing a better user experience and preventing potential issues with invalid file uploads.
Advantages of file extension checking in JavaScript
- Prevents uploading of incompatible file types.
- Helps to avoid security breaches by ensuring that only intended files are uploaded.
- Reduces server load by avoiding unnecessary uploads.
Disadvantages of file extension checking in JavaScript
- File type checking can be bypassed by malicious users using custom scripts or tools.
- JavaScript file type checking is not a substitute for server-side file type validation.
Best practices of file extension checking before uploading
- Use both client-side (JavaScript) and server-side validation to ensure that only the intended files are uploaded.
- Use server-side validation as the primary method of file type checking to prevent malicious users from bypassing client-side validation.
- Keep a list of allowed file types and regularly update it.
Checking the file type before uploading is an essential security and error-prevention measure for web applications. While JavaScript can help with client-side validation, server-side validation is still the best practice. By implementing both client-side and server-side validation, web developers can create more secure and robust web applications.
Still you face problems, feel free to contact with me, I will try my best to help you.