PHP image upload with validation
- Last Updated : 31/07/2021
Uploading an image using PHP is easy but proper validation like image type validation is a tricky one. In this post, we will learn how to upload an image with type, width, height, and size(MB, Kb) validation using PHP with free source code.
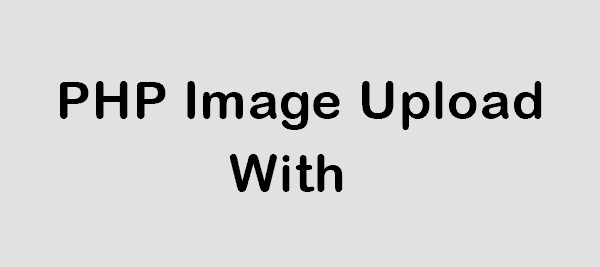
- β Basic Idea of uploading an image using PHP.
- β PHP Image type validation.
- β PHP Image width & height validation.
- β PHP Image size(MB,Kb) validation.
Basic Idea of "uploading an image using PHP".
First of all, if you want to upload an image using PHP and HTML form you need to use the action="your-script-link", the enctype="multipart/form-data" and method="post" as the form attribute. Make sure you give a proper name to your input filed.
<form action="script/upload.php" method="post" enctype="multipart/form-data"> <label>Upload File</label> <input required type="file" name="my_file"> <input type="submit" name="upload_btn"> </form>
Note: Without enctype="multipart/form-data" you can't upload file.
In your php script, you need to catch the file using PHP superglobal variable $_FILES. So first of all get the file and from the file, get the temporary path and actual name of the file.
$file = $_FILES['my_file']; $name = $file['name']; $temporary_file = $file['tmp_name']; // temporary path
Note: Don't forgot to take temporary file.
php file upload
Then use PHP built-in move_uploaded_file() function to upload the file. This function will take two parameters one this the temporary path of your image and another one is the location(with file name) where you want to upload.
$upload_location = "../upload/"; // targeted location $location_with_name = $upload_location.$name; // like upload/images/my-image.jpg move_uploaded_file($temporary_file, $location_with_name);
Note: If you want to make a unique name of your uploading image, you can use the PHP time() function when rename.
PHP makes the name unique before upload.
$str_to_arry = explode('.',$name); $extension = end($str_to_arry) // get extension of the file. $upload_location = "../upload/"; // targeted location $new_name = "upload-image-".time().".".$extension; // new name $location_with_name = $upload_location.$new_name; // finel new file move_uploaded_file($temporary_file, $location_with_name);
Note: Here we used explode function to get the extension.
PHP get file extension
$str_to_arry = explode('.',$name); $extension = end($str_to_arry) // get extension of the file.
Note:here we Used the PHP string explode() function to get the file extension. But remember to detect the actual file type you need to check the file mime type.
PHP get file mime type
$finfo = finfo_open(FILEINFO_MIME_TYPE); $file_type = finfo_file($finfo, $temporary_file);
PHP Image type validation
To validate image type you may use the file extension checking or file type technique, but checking only the extension is not the valid way to file type validation or image type validation.
Suppose you are trying to validate an image using only the file extension checking technique. Now someone uploads another type(.md) of the file (virus or hacking) just renaming the file type from .md to .jpg, in this case, your validation will be failed. So donβt apply only file type checking.
$file_type = $file['type']; if($file_type != "jpg" || $file_type != "jpeg"){ echo "This is not an image"; }
Note: Don't do this.
So the actual way to validate your file or an image using PHP is by checking file MIME-type. PHP provides a default function to check the file MIME Type.
$finfo = finfo_open(FILEINFO_MIME_TYPE); $file_type = finfo_file($finfo, $temporary_file); if($file_type != "image/jpeg" || $file_type != "image/png"){ echo "This is not an image"; }
Note: Do like this.
Note: Download the complete source code for better understanding.
PHP get image height and width
Validate image width and height using PHP is so easy, using the default PHP function getimagesize() we can easily get the width and height of the image, this method will take the temporary path of the image.
$data = getimagesize($temporary_file); $width = $data[0]; // in pixel $height = $data[1]; // in pixel if($width != 300 && $height != 300){ echo "Please Upload 300x300 pixel Image."; }
Note: Download the complete source code for better understanding.
PHP Image size validation
Validate the size(MB, kb, byte) of an image using PHP is also so easy task.
$size = $file['size']; // size in byte $mb_2 = 2000000; if($size > $mb_2){ echo βFile is too large, Upload less than or equal 2MBβ; }
Note: Download the complete source code for better understanding.
Still you face problems, feel free to contact with me, I will try my best to help you.