Sign In and Sign Up Form Using HTML CSS and JavaScript - Design 1
Last Updated: 2025-01-19 20:08:50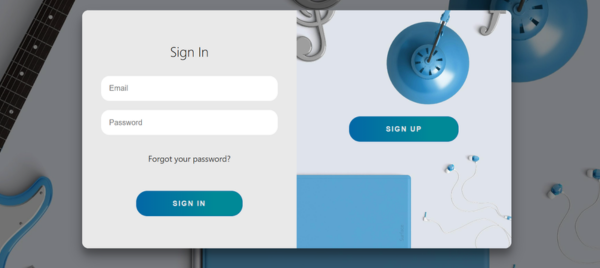
In this tutorial, we’ll create a Sign In and Sign Up form with a seamless user interface. The form is built with HTML, styled beautifully with CSS, and made interactive using JavaScript. This project is ideal for beginners looking to enhance their front-end development skills.
The project features a visually appealing Sign In and Sign Up form on a single page. A sliding panel animates between the two forms, offering a smooth transition. The Sign Up form collects user details like username, email, and password, while the Sign In form requires email and password. JavaScript is used to handle form toggling and basic interactivity.
Key Features:
- A sleek background with an overlay effect for aesthetics.
- Animated transitions between forms for a dynamic experience.
- Mobile-friendly design with CSS variables for easy customization.
Main Logic Explanation
The main logic in this project lies in switching between the Sign In and Sign Up forms. Let’s break it down by explaining the JavaScript, HTML, and CSS.
1. JavaScript Logic
This is the key logic that toggles the forms by adding or removing a CSS class (right-panel-active).
const signInBtn = document.getElementById("signIn");
const signUpBtn = document.getElementById("signUp");
const container = document.querySelector(".container");
signInBtn.addEventListener("click", () => {
container.classList.remove("right-panel-active");
});
signUpBtn.addEventListener("click", () => {
container.classList.add("right-panel-active");
});
Explanation:
- Event Listeners: When the "Sign In" or "Sign Up" buttons are clicked, the corresponding event listener triggers.
- Toggling Classes:
- container.classList.add("right-panel-active") makes the Sign Up form visible and slides the overlay panel.
- container.classList.remove("right-panel-active") brings the Sign In form to focus.
2. HTML Structure
The HTML is structured with two form containers (container-signin and container-signup) and an overlay panel to create the dynamic switching effect.
< div class="container right-panel-active">
< !-- Sign Up Form -->
< div class="container-form container-signup">
< form action="#" class="form" id="form1">
< h2 class="form-title">Sign Up< /h2>
< input type="text" placeholder="User" class="input" />
< input type="email" placeholder="Email" class="input" />
< input type="password" placeholder="Password" class="input" />
< button class="btn">Sign Up< /button>
< /form>
< /div>
< !-- Sign In Form -->
< div class="container-form container-signin">
< form action="#" class="form" id="form2">
< h2 class="form-title">Sign In< /h2>
< input type="email" placeholder="Email" class="input" />
< input type="password" placeholder="Password" class="input" />
< a href="#" class="link">Forgot your password?< /a>
< button class="btn">Sign In< /button>
< /form>
< /div>
< !-- Overlay Panel -->
< div class="container-overlay">
< div class="overlay">
< div class="overlay-panel overlay-left">
< button class="btn" id="signIn">Sign In< /button>
< /div>
< div class="overlay-panel overlay-right">
< button class="btn" id="signUp">Sign Up< /button>
< /div>
< /div>
< /div>
< /div>
Explanation:
- Container: The outer div with the class container holds all the components and controls their layout.
- Sign Up and Sign In Forms:
- Each form is placed in a div with its own class (container-signup and container-signin).
- The id attributes (form1 and form2) link to the JavaScript logic.
- Overlay Panel:
- The container-overlay contains two buttons ("Sign In" and "Sign Up") for switching between forms dynamically.
- The container-overlay contains two buttons ("Sign In" and "Sign Up") for switching between forms dynamically.
3. CSS Animations
CSS is responsible for the smooth transitions and beautiful design.
.container {
background-color: var(--white);
border-radius: var(--button-radius);
box-shadow: 0 0.9rem 1.7rem rgba(0, 0, 0, 0.25),
0 0.7rem 0.7rem rgba(0, 0, 0, 0.22);
height: var(--max-height);
max-width: var(--max-width);
overflow: hidden;
position: relative;
width: 100%;
}
.container.right-panel-active .container-signin {
transform: translateX(100%);
}
.container.right-panel-active .container-signup {
opacity: 1;
transform: translateX(100%);
z-index: 5;
}
.overlay {
background: url("bg-img.jpg") center / cover;
height: 100%;
left: -100%;
transform: translateX(0);
transition: transform 0.6s ease-in-out;
width: 200%;
}
Explanation:
- Container Transitions:
- The right-panel-active class shifts the Sign In form out of view and brings the Sign Up form into focus with smooth animations.
- The transform property handles sliding effects, while opacity ensures a fade-in effect.
- Overlay Styling:
- The overlay (container-overlay) creates a sliding background effect, transitioning smoothly between the Sign In and Sign Up buttons.
- The overlay (container-overlay) creates a sliding background effect, transitioning smoothly between the Sign In and Sign Up buttons.
Extra Tips
- Use CSS Variables: Adjust the theme by editing variables like --blue and --lightblue in the CSS file.
- Improve User Experience: Add hover effects for buttons using :hover in CSS.
- Simplify Mobile Responsiveness: Use media queries to optimize the layout for smaller screens.
Challenge Block
Now that you’ve built the Sign In and Sign Up form, try extending it further:
- Add validation for fields like email and password.
- Introduce a feature to toggle password visibility.
- Add social login buttons for platforms like Google and Facebook.
Example: “What if your users could log in with a single click? Add social media login buttons and style them to match the theme!”
This dynamic Sign In and Sign Up form is a great example of combining functionality with aesthetics. You’ve learned how to create sliding animations, toggle form views, and style components with CSS. Challenge yourself to add more features and make this project even
better!
Still you face problems, feel free to contact with me, I will try my best to help you.