Projects With C - Student Management System Store Data in Text File
Last Updated: 2023-02-23 11:41:03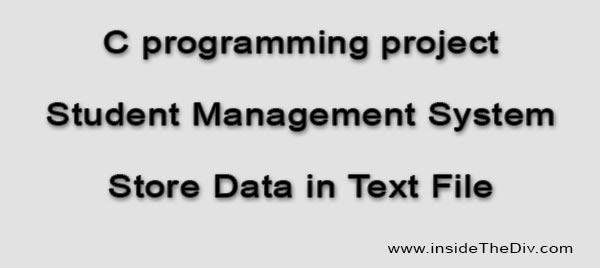
Project with c programming language is one of the best ways to learn and develop implementation skills. In this post, we will create a simple student management system project with c programming language. If you want to develop your implementation skills this project will help you a lot. We will show provide you with the complete source code so that you can apply your own logic to implement your idea.
File system project with c programming language
From the title, you already understand that this will be a file system project. We are calling this project a file system project because we will store all of the data inside the text file. So, this project will help you to understand the file handling concept of c programming language. At the end of the project, you will learn the following techniques:
- How to read and write a text file in the c programming language?
- How to edit and update a text file in the c programming language?
- How to rename a text file in c programming?
- How to delete a text file in c programming?
File System Student Management System Projects With C
In our previous example, we learned the structure array concept of c programming. So, now we will only focus on the file-handling concept of the C programming language & will try to keep our project simple. So, to keep our project we will store only two entities' information one is students and the other is course. Under the student entity, we will store students' personal information like Student ID, Name, Email, Phone, & Number of Courses. And for the course, we will store the course code and course name. Also, we will create a one-to-many relationship between the student and the course by the student ID. That means a student can have one or more courses. In real-life student management system projects, we can also create this type of relation by creating SQL tables. learn how to create a parent chile table relation in SQL.
struct StudentInfo
{
char ID[10];
char Name[20];
char Email[30];
char Phone[20];
int NumberOfCourse;
};
struct CourseInfo
{
char StudentID[10];
char Code[10];
char Name[20];
};
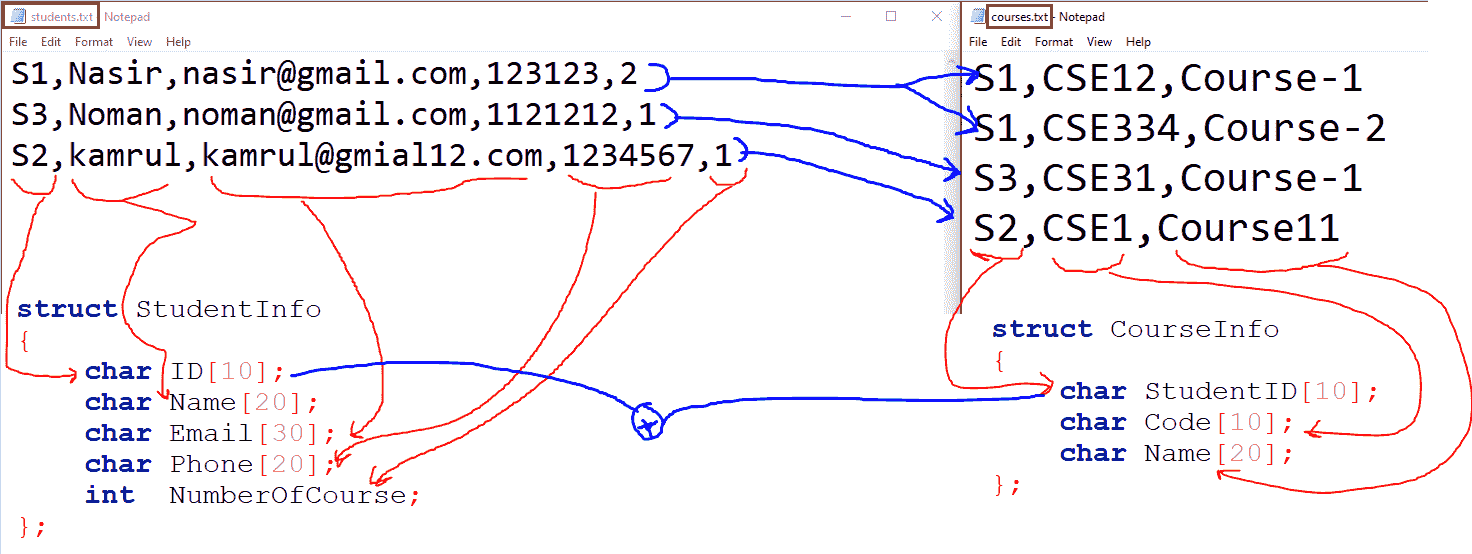
Feature of our project
As this will be a simple project we will only focus on the CRUD(Create, Read, Update & Delete) operation, so this project will have the following features
- Create a new student and store the data in a text file.
- Read all students' information from the text file.
- Search a student's information from the text file.
- Edit and Update the student's information in the text file.
- Delete a student's information from the text file.
- Delete All students' information from the text file.
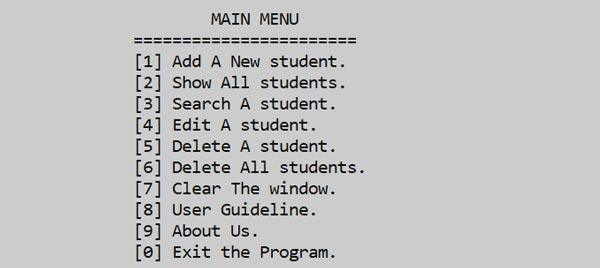
You already know that we will create a relationship between the student and the course so every feature of the student is related to the course, for example, if a user searches for a student we will show the student's course information along with his/her personal information.
For a better understanding please download the complete source code.
Create a new student and store the data in a text file
To create a student we will take the student's information from the user. For example, we will tell the user to give input the student id, name, email, phone, and number of courses. After taking all the information we will store it in our student.txt file.
Please note that we will perform some basic validation before storing the data in the text file. For example, we will check the max and min length of the name, email, and phone number also we will check if StudentID, Email & phone number are unique or not.
Here is an example code to store student information in the text file.
void AddNewStudent()
{
struct StudentInfo NewStudent;
scanf("%s",&NewStudent.ID);
scanf(" %[^\n]s",&NewStudent.Name);
scanf("%s",&NewStudent.Email);
scanf("%s",&NewStudent.Phone);
scanf("%d",&NewStudent.NumberOfCourse);
// we will perform some basic validation before storing the data in the text file.
// So please download the complete souce code.
// store information in file
FILE *file_students = fopen("students.txt", "a");
fprintf(file_students, "%s,%s,%s,%s,%d\n",NewStudent.ID,NewStudent.Name,NewStudent.Email,NewStudent.Phone,NewStudent.NumberOfCourse);
fclose(file_students);
// end
}
The AddNewStudent function is a program that adds information about a new student to a text file. The function takes in input from the user about a student's ID, name, email, phone number, and number of courses, stores this information in a struct named StudentInfo, and then writes this information to a text file named "students.txt".
The information is written to the text file in a comma-separated format, with each line representing the information for a single student. The function opens the text file in append mode, which allows it to add new information to the end of the file without overwriting any existing data.
By calling this function, the program provides a way to store and manage information about students in a simple and organized manner.
For a better understanding please download the complete source code.
Read all students' information from the text file.
To show all students' information we will create a separate function for example we will create the "show_all_students" function is used to display the information of all the students stored in the "student.txt" file. The function opens the file and reads the data line by line using the fscanf function. The student information is separated by commas and each line represents a single student's information. The function then uses printf statements to print the information of each student on the console.
Please note that we will show all information in a table format, so please download the complete source code.
void ShowAllStudents()
{
char line[MAX_LINE_LENGTH];
char *delimiter = ",";
char *ID;
char *Name;
char *Email;
char *Phone;
char *NumberOfCourse;
FILE *StudentFile = fopen("students.txt","r");
while(fgets(line,MAX_LINE_LENGTH,StudentFile)){
ID = strtok(line,delimiter);
Name = strtok(NULL,delimiter);
Email = strtok(NULL,delimiter);
Phone = strtok(NULL,delimiter);
NumberOfCourse = strtok(NULL,delimiter);
printf("%s\n",ID);
printf("%s\n",Name);
printf("%s\n",Email);
printf("%s\n",Phone);
printf("%s\n",NumberOfCourse);
// we will show in a table format
// so download the complete souce code
}
fclose(StudentFile);
}
The ShowAllStudents function is a program that retrieves information about all the students from a text file named "students.txt" and displays the information on the screen.
The function opens the text file using fopen and reads it line by line using a while loop and the fgets function. Each line represents the information for a single student, stored in a comma-separated format. The function uses the strtok function to extract each field of information from each line.
The extracted information is then displayed on the screen using printf, with each field of information displayed on a separate line. The file is closed using fclose after all the information has been retrieved and displayed.
By calling this function, the program provides a way to view all the information about students stored in the "students.txt" file in a simple and organized manner.
For a better understanding please download the complete source code.
Search a student's information from the text file.
In our project, we will create a search option so that users can search for students by their student ID.
The "search_student" function is used to find and display information about a particular student based on the student ID. The function takes the student ID as input and then opens the "student.txt" file to read the data line by line. The function uses the fscanf function to read the data and the strcmp function to compare the student ID in each line with the input student ID.
If a match is found, the function prints the information of the student on the console using printf statements. If the student ID is not found, the function displays a message indicating that the student was not found. Please note that we will also show the course information from the course.txt file. So, please download the complete source code.
The function can be written as follows:
void SearchStudent(char StudentID[10])
{
FILE *studentsFile = fopen("students.txt", "r");
while(fgets(line,MAX_LINE_LENGTH,studentsFile))
{
ID = strtok(line,",");
if(strcmp(ID,StudentID) == 0)
{
// we found a student
found = true;
printf("\n One Student Found for ID: %s\n\n",StudentID);
printf(" Student Informations\n");
printf("-------------------------\n");
printf(" ID: %s\n",ID);
Name = strtok(NULL,","); // name
printf(" Name: %s\n",Name);
Email = strtok(NULL,","); // email
printf(" Email: %s\n",Email);
Phone = strtok(NULL,","); // phone
printf(" Phone: %s\n",Phone);
NumberOfCourse = strtok(NULL,","); // number of courses
printf(" Number of Courses: %s\n",NumberOfCourse);
break;
}
}
fclose(studentsFile);
// we will aslo show the student course information.
// so please download the complete source code.
}
The SearchStudent function is a program that searches for a student based on their ID and displays the information for that student on the screen.
The function takes as an argument a string, StudentID, which is the ID of the student to search for. The function then opens the text file named "students.txt" using fopen and reads it line by line using a while loop and the fgets function.
Each line represents the information for a single student, stored in a comma-separated format. The function uses the strtok function to extract the student ID from each line. If the extracted ID matches the StudentID argument, the function sets the found variable to true and retrieves the information for that student by calling strtok multiple times.
The extracted information is then displayed on the screen using printf, with each field of the information displayed on a separate line. The file is closed using fclose after either the student information has been retrieved and displayed or the end of the file has been reached.
By calling this function and passing in a student ID as an argument, the program provides a way to search for and view the information for a specific student stored in the "students.txt" file.
For a better understanding please download the complete source code.
Edit and Update the student's information in the text file.
Updating a student's information in the text file is one of the hard parts of this project. We will create a separate function for editing and updating students' information.
The "edit_student" function is used to update the information of a particular student. The function takes the student ID as input and then opens the "student.txt" file to read the data line by line. The function uses the fscanf function to read the data and the strcmp function to compare the student ID in each line with the input student ID.
If a match is found, the function allows the user to update the information of the student by calling appropriate functions to update each field (e.g. update_name, update_email, etc.). The updated information is then stored in a temporary file, which is created using the fopen function.
Once all the updates are complete, the function closes the original file and renames the temporary file to the original file name. This effectively updates the original file with the new information.
The concept of a temporary file is used in this function because it allows the program to make changes to a file without modifying the original file directly. This is useful in case something goes wrong during the update process, as the original file can be preserved.
Steps we will follow
- Create a temp.txt file
- Copy all students from the main file(student.txt) to the temp file, except your targeted student.
- Get updated information from the user.
- Store updated the information in the temp file
- Delete the main file.
- Rename the temp file as the main file(from temp.txt to student.txt).
Here is an example code to update student information in a text file. But please note that in our project we have added some extra features like the user will able to skip editing and the user will able to update the course information. So please download the complete source code. In our previous example, we have also added this type of feature you can check out this also.
void EditStudent(char StudentID[10])
{
// copy main to temp
FILE *MainStudentsFile = fopen("students.txt", "r");
FILE *TempStudentsFile = fopen("temp-students.txt", "w");
while(fgets(line,MAX_LINE_LENGTH,MainStudentsFile)){
strcpy(line2,line);
old_ID = strtok(line,delimiter);
if(strcmp(old_ID,StudentID) != 0)
{
fprintf(TempStudentsFile,"%s",line2);
}
}
// get updated data
struct StudentInfo UpdatedStudent;
scanf(" %[^\n]s",&UpdatedStudent.Name);
scanf("%s",&UpdatedStudent.Email);
scanf("%s",&UpdatedStudent.Phone);
scanf("%d",&UpdatedStudent.NumberOfCourse);
// store updated data to temp
// added updated student to our temp file
fprintf(TempStudentsFile, "%s,%s,%s,%s,%d\n",old_ID,UpdatedStudent.Name,UpdatedStudent.Email,UpdatedStudent.Phone,UpdatedStudent.NumberOfCourse);
fclose(TempStudentsFile);
fclose(MainStudentsFile);
remove("students.txt"); // delete main
rename("temp-students.txt","students.txt"); // rename temp to main
// end
}
This code implements the functionality to edit the information of a student record in a text file named "students.txt". The function takes in a parameter StudentID which is the identifier of the student whose information needs to be updated.
- The code first opens two files, the original file "students.txt" in read mode and a temporary file "temp-students.txt" in write mode.
- It then reads each line of the original file using fgets and strtok to extract the student ID from the line. If the extracted ID is not equal to the StudentID passed as a parameter, it writes the entire line to the temporary file.
- Next, the code prompts the user to input the updated information for the student, and stores it in a struct named UpdatedStudent.
- The code then appends the updated student information to the temporary file, and closes both the original and the temporary file.
- Finally, the original file is deleted and the temporary file is renamed to "students.txt", effectively replacing the original file with the updated information.
For a better understanding please download the complete source code.
Delete a student's information from the text file.
If you understand the editing concept the deleting concept will be an easy task for you. Because deleting students' information from a text files is the same as editing, you just need to follow the following steps.
- Create a temp.txt file
- Copy all students from the main file(student.txt) to the temp file, except your targeted student.
- Delete the main file.
- Rename the temp file as the main file(from temp.txt to student.txt).
For a better understanding please download the complete source code.
Delete All students' information from the text file.
Deleting all student information from the text file is so easy task you just need to delete the main file and create an empty file again.
void DeleteAllStudents()
{
remove("students.txt");
FILE *TempStudentsFile = fopen("students.txt", "w");
fclose(TempStudentsFile);
remove("courses.txt");
FILE *TempCoursesFile = fopen("courses.txt", "w");
fclose(TempCoursesFile);
printf(" All Students Deleted Successfully.\n\n");
}
The function DeleteAllStudents removes two files: "students.txt" and "courses.txt". The file "students.txt" is removed using the remove function and then recreated as an empty file using fopen and fclose functions. The file "courses.txt" is also deleted using remove and recreated as an empty file using fopen and fclose functions. Finally, it prints a message "All Students Deleted Successfully."
For a better understanding please download the complete source code.
Conclusion
This is a simple project with c programming where we have focused only on the file-handling process by adding edit and deleting student information inside a text file. Overall to create a student management system project with c where you want to store student information inside the text file you can follow this post. Also, we have provided the complete source code and video so that you can easily understand the techniques.
Still you face problems, feel free to contact with me, I will try my best to help you.