Create and Download zip file in PHP code
- Last Updated : 21/08/2021
Creating a zip file or downloading all files as zip files using PHP is not so hard. You just need to create a zip file using the PHP touch() function then add all files to the created zip file and finally automatically download the file using the header content.
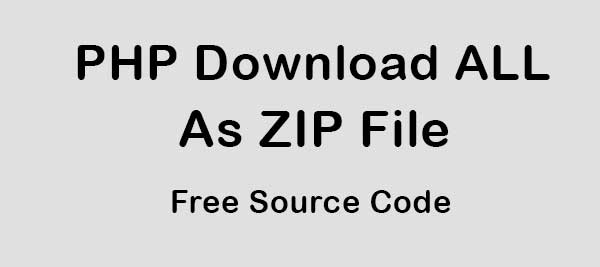
In this post, I will provide you a complete guideline for.
- How to create a zip file in PHP?
- How to add files to zip in PHP?
- How to download zip in PHP?
PHP create zip file on server
Creating a file or creating any file is exactly the same in PHP you just need the default PHP touch() function. This function will take the file name with location and return you a file.
$zip_file_name_with_location = "/zip-file/all-image.zip"; touch($zip_file_name_with_location); // just create a zip file
PHP add files to zip
Now if you want to add or insert some files into your zip file you need to open your zip file under the default ZipArchive class using the open() methods. This method will take your zip file name with location. After opening your zip file then insert your files into the opened zip file using the addFile() method, this method will take two parameters one is the file name with the location(which you want to insert) and another is the file name without location. After adding all files you need to close your opening zip file.
$zip = new ZipArchive; $opening_zip = $zip->open($zip_file_name_with_location); // add a image to this opening zip file $opening_zip->addFile($inserting_file_name_with_location,$inserting_file_name_without_location); $opening_zip>close();
Note: suppose you want to rename your inserting file name inside the zip file then you can do it just using your accepted name as a $inserting_file_name_without_location.
Let,
your inserting file name with location is “/images/ngo-2342341.jpg”
And,
without location, the file name is “ngo-2342341.jpg”.
You want to add this file as “image-1.jpg”.
So you can do it like this.
$opening_zip->addFile($inserting_file_name_with_location,”image-1.jpg”);
In this example, we just add or insert one file to our zip file, but to add all files of a folder you need to fetch all files from a folder.
PHP download zip file from server
To download your zip file automatically you just need to set some header content-type and content description then read the file.
// file will download ad this name $demo_name = "my-image.zip"; header('Content-type: application/zip'); header('Content-Disposition: attachment; filename="'.$demo_name.'"'); readfile($zip_file_name_with_location); // auto download //if you wnat to delete this zip file after download unlink($zip_file_name_with_location);
Still you face problems, feel free to contact with me, I will try my best to help you.