Click on the image to view full size in a popup modal
- Last Updated : 17/06/2021
Viewing the full-size image in a modal after clicking on the thumbnail, is one of the cool features of every website. In this post, we will learn how can create "onclick popup image" features using HTML CSS and jquery. Also, I will provide you the complete source code.
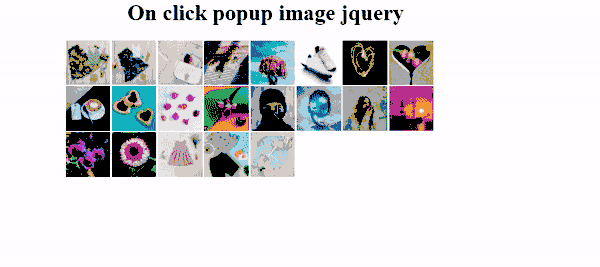
Download the complete soruce code free!
So to create the onclick popup image modal we need to follow some steps
- 👉 Create a popup modal to view a full-size image after the click.
- 👉 Get and set image src from thumbnail image to modal image.
- 👉 Add and remove an active class to the thumbnail image.
Create a popup modal to view full-size image after the click
To view an image in full size in a popup modal first we need to create a modal using HTML and CSS javascript or jquery. We can use jquery or javascript but to give some animation easily in our popup modal we need to use jquery.
The HTML structure of popup modal
We already decided that we will show our image on a popup modal, which means our modal will contain an image tag. Also, we will take a close button so that we can close the modal.
<!-- // popup modal --> <div id="show_image_popup"> <div class="close-btn-area"> <button id="close-btn">X</button> <!-- close btn --> </div> <div id="image-show-area"> <img id="large-image" src="" alt=""> <!-- popup imge --> </div> </div>
Desing a popup modal using CSS
To design our modal we will use CSS position and z-index property so that our modal does not take place in the website’s main content. To keep the modal hide for the first time we will use CSS display none property.
#show_image_popup{ position: absolute; /* so that not take place */ top: 50%; left: 50%; z-index: 1000; /* adobe all elements */ transform: translate(-50%, -50%); /* make center */ display: none; /* to hide first time */ } #show_image_popup img{ max-width: 90%; height: auto; }
Download the complete soruce code free!
Show popup modal after clicking on the image using jquery.
To show our popup modal we can use the jquery show() method. But to show popup modal with animation we can use the fadeIn() method.
$(".small-image").click(function(){ // $("#show_image_popup").fadeIn(); $("#show_image_popup").show() })
hide popup modal after clicking on the close button using jquery
To hide our popup modal we can use the jquery hide() method. But to hide popup modal with animation we can use the fadeOut() method.
$(".close-btn").click(function(){ // $("#show_image_popup").fadeOut() $("#show_image_popup").hide() })
Note:
- 👉 You can also hide the modal automatically with a time countdown.
- 👉 If you are familiar with bootstrap you can use the bootstrap modal witch is more easy and smart design.
Get and set image src from thumbnail image to modal image
To create an “onclick popup image modal” the main idea is getting and setting the src attribute of the image tag. We know we have an image tag on our popup modal so when we will click on our thumbnail image we will get the value of its src attribute then we will set this value to our modal’s image tag.
How to get image src attribute value in Jquery
Getting an image’s src value in jquery is not like the raw javascript we need to use the attr() method. This method will take the attribute name as a string.
var src_value = $("#my_img"”).attr('src');
How to set image src attribute value in Jquery
To Set an image’s src value in jquery we also need to use the attr() method, this time the attr() method will take two parameters one the attributes name and another one is the value you want to set.
Var src_value = “my-image.jpg”; $("#modal_img ").attr('src',src_value);
Add and remove an active class to the thumbnail image using jquery
After clicking on the thumbnail image, we need to make the image active by adding an active class. So that you can understand which thumbnail image is currently viewed. In jquery to add a class, we need to use the addClass() method and to remove we need to use the removeClass() method.
Note:
- 👉 Make sure you design your active class
- 👉 Also, make sure you remove the class when you close the modal and click on another image.
.active{ filter: blur(5px); }
$(".small-image").click(function(){ $(this).addClass('active'); // add active class $(".small-image").removeClass('active'); // remove active class from all images })
Download the complete soruce code free!
Logical code of onclick popup image
$(".small-image").click(function(){ removeActiveClassFromAllImage(); // this function will remove all image from all small images addActiveClass(this_image); // this function will add active class to this image getImageSrc(this_img); // this function will get the src value from this small image setSrctoModalImage(this_img_src); // this function will set src to the modal image tag showModal(); // will show our modal }) $(".close-btn").click(function(){ removeActiveClassFromAllImage(); // will remove all active class closeModal(); // will close our modal })
Watch the video or download the complete source code for free.
Still you face problems, feel free to contact with me, I will try my best to help you.