Javascript filter table row single and multiple columns
- Last Updated : 11/12/2021
Filtering data from a table using javascript is a common and effective feature of every web application. Because it is fast and easy to implement. In this post, I will show you how can you develop javascript filter table features in your project.
Why javascript table filter is fast?
You may already know javascript is a client-side language, so if you use javascript to filter data from the table the process will be fast because javascript will execute on your browser and you do not need to send any request to the server.
Algorithm of the javascript table filter feature
The steps of javascript table filter implementation are not so hard, you just need to check if your targeted column value contains the characters you typed on the search box. If it contains then show only thous rows and hide other rows.
- Take the search box value using javascript onKeyUp event.
- Select all rows using getElementByTagName
- Run a loop to all the rows
- For every single row select your targeted column just like the row selection
- Check the column value contains your search keyword or not using the javascript indexOf method.
- If the indexOf method return a positive value shows the row otherwise hide the row
a. Add inline CSS display property to show and hide
Table Design For Search In HTML Table Using Javascript
Designing a table for this project is not so difficult thing, you can design as you want but you should keep it simple so that you can catch the text of the table's column easily. Because we will catch the text by HTML tag using javascript. Also, make sure you design a search box to type your keyword
Download the complete source code for better understanding.javascript filter table row single columns
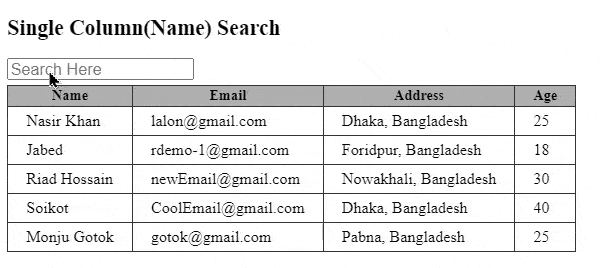
To filter table rows based on a single column first of all you need to specify your targeted column. To select your targeted column you can use javascript getElementByTagname, and to get the value of the column you can use javascript textContent or innerText. Remember the javascript getElementByTagname will return an Array os your column indexing will start from zero.
searchBox_1.addEventListener("keyup", function(){ var keyword = this.value; keyword = keyword.toUpperCase(); var table_1 = document.getElementById("table-1"); var all_tr = table_1.getElementsByTagName("tr"); for(var i=0; i<all_tr.length; i++){ var name_column = all_tr[i].getElementsByTagName("td")[0]; if(name_column){ var name_value = name_column.textContent || name_column.innerText; name_value = name_value.toUpperCase(); if(name_value.indexOf(keyword) > -1){ all_tr[i].style.display = ""; // show }else{ all_tr[i].style.display = "none"; // hide } } } });Download the complete source code for better understanding.
javascript filter table row multiple columns
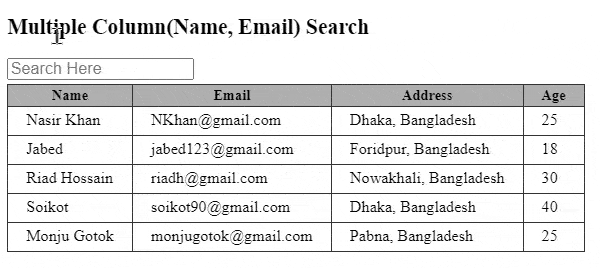
To filter table rows based on multiple columns you need to select your targeted columns just like single columns.
searchBox_2.addEventListener("keyup",function(){ var keyword = this.value; keyword = keyword.toUpperCase(); var table_2 = document.getElementById("table-2"); var all_tr = table_2.getElementsByTagName("tr"); for(var i=0; i<all_tr.length; i++){ var name_column = all_tr[i].getElementsByTagName("td")[0]; var email_column = all_tr[i].getElementsByTagName("td")[1]; if(name_column && email_column){ var name_value = name_column.textContent || name_column.innerText; var email_value = email_column.textContent || email_column.innerText; name_value = name_value.toUpperCase(); email_value = email_value.toUpperCase(); if((name_value.indexOf(keyword) > -1) || (email_value.indexOf(keyword) > -1)){ all_tr[i].style.display = ""; // show }else{ all_tr[i].style.display = "none"; // hide } } } })Download the complete source code for better understanding.
javascript filter table row all columns
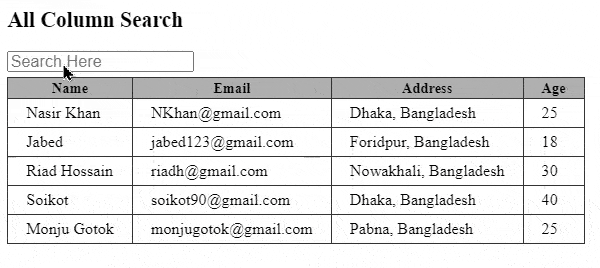
To filter table rows based all columns you need to select all columns and run a loop to all columns just like the row's loop.
searchBox_3.addEventListener("keyup",function(){ var keyword = this.value; keyword = keyword.toUpperCase(); var table_3 = document.getElementById("table-3"); var all_tr = table_3.getElementsByTagName("tr"); for(var i=0; i<all_tr.length; i++){ var all_columns = all_tr[i].getElementsByTagName("td"); for(j=0;j<all_columns.length; j++){ if(all_columns[j]){ var column_value = all_columns[j].textContent || all_columns[j].innerText; column_value = column_value.toUpperCase(); if(column_value.indexOf(keyword) > -1){ all_tr[i].style.display = ""; // show break; }else{ all_tr[i].style.display = "none"; // hide } } } } })
Still you face problems, feel free to contact with me, I will try my best to help you.