How to add class to element JavaScript - add active Class on Menu
Last Updated: 2023-07-31 05:48:06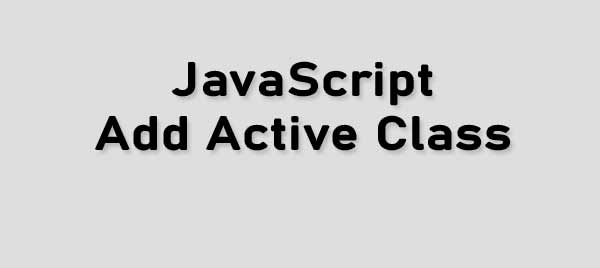
This is javascript's onclick event-related post. In this post, we will learn how to add a class to an HTML element in javascript. We will add an active class to a menu when a user clicks on a menu item. We will also give you the complete source code so that you can implement your own logic easily.
By the end of this post will learn the following concepts:
- How to add a class to an HTML element
- How to add a class to the current elements
- How to make a menu active when the user clicks on it
Add class to an HTML Element JavaScript
Adding a class to an HTML element in javascript is so easy. You just need to select your targeted element and then change its attributes. Let's assume you have an HTML button element and you want to change its class using javascript. Now you need to follow the following steps:
- Retrieve the button element using JavaScript. You can use javascript getElementByID to get the button.
- Get the class list and add your new class.
var button = document.getElementById('myButton');
button.classList.add('newClass');
Here the myButton is your button's ID and the newClass is the class you want to add to your button.
How to add a class to the current elements
If you know how to add a class to an HTML element then adding a class to the current element is nothing for you. Let's assume you have three HTML buttons and you want to add a class to the current button you clicked on. So now you need to follow the following steps.
Step 1: Add an event listener to each button to detect when it is clicked. You can do this by selecting the buttons using a class. Let's assume all the buttons have a class called “myButton”
var buttons = document.getElementsByClassName('myButton');
for (var i = 0; i < buttons.length; i++) {
buttons[i].addEventListener('click', function() {
// Step 2 goes here
});
}
Step 2: Inside the event listener function, you can access the clicked button using the ‘this’ keyword. You can then add a class to it using the classList.add() method.
var buttons = document.getElementsByClassName('myButton');
for (var i = 0; i < buttons.length; i++) {
buttons[i].addEventListener('click', function() {
this.classList.add('newClass');
});
}
In the above code, when a button is clicked, the "newClass" is added to that specific button, indicating that it has been clicked.
How to make a menu active when the user clicks on it
If you know how to add a class to the current element then making a menu active is easy for you. You just need to design an active class in CSS and then add this class to your element when the user clicks on the menu. Let's assume you have a menu with 5 links and you have an active class in your CSS file. Now you want to add this class to your current menu item to make it active. So, you can follow the following steps.
Step 1: Add an event listener to each menu item to detect when it is clicked. You can select the menu items using a class, id, or any suitable method. Here, we'll assume the menu items have a class called "menu-item".
.menu-item {
/* Styles for the menu items */
/* Add your desired styles here */
}
.menu-item.active {
/* Styles for the active menu item */
/* Add your desired styles here */
}
var menuItems = document.getElementsByClassName('menu-item');
for (var i = 0; i < menuItems.length; i++) {
menuItems[i].addEventListener('click', function() {
// Step 2 goes here
});
}
Step 2: Inside the event listener function, you can remove the "active" class from all menu items and then add the "active" class to the clicked menu item.
var menuItems = document.getElementsByClassName('menu-item');
for (var i = 0; i < menuItems.length; i++) {
menuItems[i].addEventListener('click', function() {
// Remove "active" class from all menu items
for (var j = 0; j < menuItems.length; j++) {
menuItems[j].classList.remove('active');
}
// Add "active" class to the clicked menu item
this.classList.add('active');
});
}
In the above code, when a menu item is clicked, the "active" class is removed from all menu items using a loop. Then, the "active" class is added to the clicked menu item using this.classList.add('active'), indicating that it is the currently active menu item.
Make sure you have defined the styles for the "active" class in your CSS file to visually indicate the active state of the menu item.
Still you face problems, feel free to contact with me, I will try my best to help you.