JavaScript Simple Projects With Source Code - Speed Calculator
Last Updated: 2023-06-11 04:04:13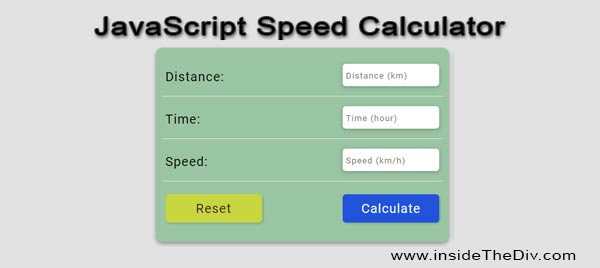
Developing a javascript simple project is one of the best ways to learn javascript. As a beginner, by developing some javascript simple projects, you can start learning javascript. In this post, we will discuss how to create a simple speed calculator in javascript we will also provide you the complete source code.
This project involves creating a simple form that allows users to enter the distance traveled and the time taken and then calculate the speed in kilometers per hour (km/h). In this project, you will learn how to create a basic HTML form, capture user input using JavaScript, and perform simple calculations using JavaScript. By the end of the project, you will have a fully functional simple speed calculator that you can use to calculate the speed of any given input.
JavaScript Projects For Beginners With Source Code
If you are a beginner in javascript we are suggesting you develop some simple projects using the basic functionality of javascript. Here are some simple javascript projects for beginners with source code. Go through each link and read the technique. Also, if you want you can download the complete source code.
- Javascript Tip calculator
- Javascript Temperature Converter
- Javascript Length Converter
- Javascript Percentage Calculator
- Javascript Fraction Calculator
- Javascript BMI Calculator
- Javascript Speed Calculator
- Javascript Online Stopwatch Full Screen
- Javascript Online Timer
- Javascript Online Digital Clock
What is a Speed Calculator?
A speed calculator is a tool that calculates the speed of an object or vehicle based on the distance traveled and the time taken to cover that distance. The speed is typically measured in units such as kilometers per hour (km/h), miles per hour (mph), or meters per second (m/s).
As our target is creating a javascript simple project so we will only focus on the main logical part here. But don't worry we will provide you with the complete source code.
JavaScript Simple Projects Speed Calculator
A JavaScript speed calculator is a program written in the JavaScript programming language that takes inputs such as distance and time, and calculates the speed based on those inputs. The speed calculator takes the distance traveled and the time taken, and uses the formula:
Speed = Distance / Time
The calculator will perform the necessary calculations and provide the result, which represents the speed at which an object or person has traveled.
Use of a Speed Calculator
A speed calculator can be useful in a variety of real-life situations. Here are six examples of how a speed calculator can be used:
- Road trips: When planning a road trip, it can be helpful to calculate the average speed you will need to maintain to reach your destination on time. You can use a speed calculator to determine the average speed you need to maintain over a given distance.
- Running: Runners often use a speed calculator to determine their running pace, which is the time it takes to run a certain distance. This can be helpful for tracking progress and setting goals.
- Cycling: Cyclists can use a speed calculator to determine their speed while cycling, which can help them track their progress and set goals for increasing their speed.
- Aviation: Pilots use a speed calculator to determine the groundspeed of their aircraft, which is the speed of the aircraft relative to the ground. This information can be used to determine the time it will take to reach a destination and to plan fuel consumption.
- Racing: Athletes who participate in races such as track and field events or car races use a speed calculator to determine their speed during the race, which can help them make strategic decisions during the race.
- Weather: Meteorologists use a speed calculator to calculate wind speed and direction, which is an important factor in predicting weather patterns and making weather forecasts.
Overall, a speed calculator is a useful tool for anyone who needs to calculate speed in various situations, whether for work or personal purposes.
Design the JavaScript Simple Projects speed calculator in HTML CSS
First, we need to create an HTML layout then we will design the layout with CSS,
Here are the steps to design a simple speed calculator for the web using HTML, and CSS:
- Create a new HTML file and add a form element with input fields for distance and time, as well as a submit button.
- Add a div element to display the result of the calculation, such as the speed in km/h.
- In the CSS file, style the form and result div element to make them visually appealing.
- In the JavaScript file, add an event listener to the submit button to capture the form data when the user submits the form.
- Inside the event listener, calculate the speed in km/h by dividing the distance by the time.
- Update the text content of the result div element with the calculated speed.
- Test the speed calculator by entering values into the form and submitting it to see the result.
We will create an HTML form that consists of two input fields for distance and time, a button to calculate the speed, and a result field to display the calculated speed.
< div>
< label for="distance">Distance (in meters)< /label>
< input type="number" id="distance" name="distance" required>
< /div>
< div>
< label for="time">Time (in seconds)< /label>
< input type="number" id="time" name="time" required>
< /div>
< button type="button" onclick="calculateSpeed()">Calculate Speed< /button>
< div>
< label for="result">Result (in meters per second)< /label>
< input type="text" id="result" name="result" readonly>
< /div>
In the above HTML code, we have two input fields for distance and time with a required attribute to ensure that the user enters a value in these fields. We also have a button that triggers the calculateSpeed() function and a result field to display the calculated speed. The read-only attribute ensures that the user cannot edit the result field.
Please note that this is a simple design of a speed calculator you can download a complete professional version from here.
We will add some CSS to style our form and center it on the page.
form {
display: flex;
flex-direction: column;
align-items: center;
margin-top: 50px;
}
label {
margin-right: 10px;
}
input[type="number"] {
width: 100px;
padding: 5px;
border: 1px solid #ccc;
border-radius: 4px;
}
button {
padding: 10px;
background-color: #4CAF50;
color: white;
border: none;
border-radius: 4px;
cursor: pointer;
}
button:hover {
background-color: #3e8e41;
}
input[type="text"] {
width: 100px;
padding: 5px;
border: 1px solid #ccc;
border-radius: 4px;
}
In the above CSS code, we have added styles to our form to make it look better. We have added padding, borders, and background color to our input fields and button. We have also centered our form on the page using flexbox.
Please note download a complete professional version from here.
JavaScript Simple Projects Speed Calculator Example Code
Suppose a car travels a distance of 150 kilometers in 2.5 hours. To calculate the speed of the car in km/h, we can use the formula:
Speed (km/h) = Distance (km) / Time (h)
Substituting the given values, we get:
Speed (km/h) = 150 km / 2.5 h = 60 km/h
Therefore, the speed of the car is 60 km/h.
Now, here are the steps to create a JavaScript function that calculates the speed:
- Create a function called calculateSpeed that takes two parameters: distance and time.
- Inside the function, calculate the speed in km/h using the formula speed = distance / time.
- Round the speed to 2 decimal places using the toFixed method.
- Return the speed as a string with the format "Speed: {speed} km/h".
- Test the function by calling it with some sample inputs and displaying the output.
Here is some sample code to get you started:
function calculateSpeed() {
// Get the distance entered by the user
var distance = parseFloat(document.getElementById('distance').value);
// Get the time entered by the user
var time = parseFloat(document.getElementById('time').value);
// Get a reference to the result field
var result = document.getElementById('result');
// Check if the time is greater than 0
if (time > 0) {
// Calculate the speed using the formula distance / time
var speed = distance / time;
// Display the speed in the result field with two decimal places
result.value = speed.toFixed(2);
} else {
// Display an error message in the result field
result.value = "Invalid input: time must be greater than 0";
}
}
This function can be integrated into a larger JavaScript program or used in conjunction with HTML and CSS to create a web-based speed calculator, as described in my previous response.
Please download the complete source code from here.
How This Project Will Help Beginners to Learn Javascript
This project will help beginners to learn JavaScript in several ways:
- DOM Manipulation: Beginners will learn how to manipulate the Document Object Model (DOM) to get and set the values of HTML elements using JavaScript.
- Event Handling: Beginners will learn how to handle user events, such as button clicks, and perform actions in response to those events.
- Variable Declaration and Scope: Beginners will learn how to declare variables in JavaScript and understand their scope, such as local and global scope.
- Conditional Statements: Beginners will learn how to use conditional statements, such as if-else statements, to control the flow of their code based on certain conditions.
- Debugging: Beginners will learn how to debug their code and fix errors using browser developer tools.
Conclusion
In conclusion, a speed calculator is a useful tool for calculating the speed of an object or vehicle based on the distance traveled and the time taken to travel that distance. With JavaScript, we can create a simple speed calculator that takes input values from the user, performs the necessary calculations, and displays the result in a user-friendly format.
Creating a speed calculator project is a great way for beginners to practice their JavaScript skills and gain experience with HTML and CSS as well. By following the step-by-step guidelines outlined above, beginners can create their own custom speed calculator and gain a deeper understanding of how JavaScript can be used to perform calculations and manipulate data on the web.
Overall, the speed calculator project is a great way to get started with JavaScript and gain confidence in your ability to create useful and interactive web applications.
Still you face problems, feel free to contact with me, I will try my best to help you.