Email validation on javascript with source code
- Last Updated : 27/08/2021
Email validation is one of the most important things when we want to collect email addresses from our users. The most common use of "email validation on javascript" is in the contact us page of any website. In this post, we will learn how email validation on javascript works.
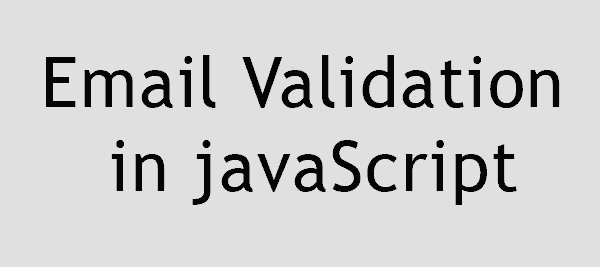
What is the valid format of an email address?
Before start validating an email we need to know the valid format of an email address. If you have a clear idea about valid email format you can easily validate an email using javascript.
Rules of valid email format
-
β
A valid email has three parts
- Local part
- Symbol part
- Domain part
- β The local part can contain a number(0,9), characters(A-Z, a,z), underscores, dashes, and dot(.).
- β Local Part also can contain special characters, such as # ! % $ β & + * β / = ? ^ _`. { | } ~
- β The maximum length of the local part is 64 characters.
- β The symbol part can contain only one symbol(@)
- β The domain part can contain at-list one dot(.) and after the dot minimum of two characters.
- β The domain part also contains numbers, characters, and dashes but one in the first place.
Some examples of the valid email address
invalid | valid |
---|---|
-9i{@-ssss.ss | -9i{@ssss.ss |
my@as@sd.com | myas@sd.ocm |
live@asd.c | live@asd.cc |
eive@a.sd.c | eive@asd.c |
Source code of email validation on javascript
Now based on these conditions you can validate your email address using javascript. You can also apply your own condition.
Email validation in javascript - Raw code
var email = βcontact@insideTheDiv.comβ; var email_length = email.length; var postion_of_at = email.indexOf('@'); var prequency_of_at = (email.split("@").length) - 1; if( email < 6){ // email min length validation console.log("Too Short Email Address") }else if(email.length > 254){ // email max length validation console.log("Too large Email") }else if(postion_of_at < 0){ console.log("@ Not Present") }else if(postion_of_at == 0){ console.log("@ present but in very First place") }else if((email_length-1) == postion_of_at){ console.log("@ present but in very Last place") }else if(prequency_of_at > 1){ console.log("Multiple @ present") }else{ console.log("Yes Valid Email") }
Note: this is just an example code, you need to apply your own condition. For real-life we suggest using regular expression.
Email validation on javascript regex
The best way of email validation on javascript is to use regex (regular expression). You can validate your email using regular expressions without using javascript just using only the HTML. If you want to keep your code simple you can do email validation using only HTML.
Regular expression for email validation
/^(([^<>()[\]\\.,;:\s@"]+(\.[^<>()[\]\\.,;:\s@"]+)*)|(".+"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\])|(([a-zA-Z\-0-9]+\.)+[a-zA-Z]{2,}))$/
function validateEmail(email) { const pattern = /^(([^<>()[\]\\.,;:\s@"]+(\.[^<>()[\]\\.,;:\s@"]+)*)|(".+"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\])|(([a-zA-Z\-0-9]+\.)+[a-zA-Z]{2,}))$/; return pattern.test(String(email).toLowerCase()); } var email = "-9i{@ssss.ss"; var is_valid = validateEmail(email); if(is_valid === true){ console.log("Yes Valid Email") }else{ console.log("Not Valid Email") }
Here the validEmail function(user-defined) will take our email as a string and match it with the regular expression. Finally, if our email matches the regular expression it will return true otherwise false. Now based on the returned value we can easily validate our email.
Still you face problems, feel free to contact with me, I will try my best to help you.